最近很多小伙伴都在问ALITTLEGUIDEONUSINGFUTURESFORWEBDEVELOPERS这两个问题,那么本篇文章就来给大家详细解答一下,同时本文还将给你拓展10BestHTML5Dev
最近很多小伙伴都在问A LITTLE GUIDE ON USING FUTURES FOR WEB DEVELOPERS这两个问题,那么本篇文章就来给大家详细解答一下,同时本文还将给你拓展10 Best HTML5 Development Tools For Web Develop...、10 HTML5 text editors for web developers and designers、100 Free Courses & Tutorials for Aspiring iPhone App Developers、8 Python Frameworks For Web Developers等相关知识,下面开始了哦!
本文目录一览:- A LITTLE GUIDE ON USING FUTURES FOR WEB DEVELOPERS
- 10 Best HTML5 Development Tools For Web Develop...
- 10 HTML5 text editors for web developers and designers
- 100 Free Courses & Tutorials for Aspiring iPhone App Developers
- 8 Python Frameworks For Web Developers
A LITTLE GUIDE ON USING FUTURES FOR WEB DEVELOPERS
Why – Or Better Web Performance by Using Futures
Performance of web applications is important to users. A web site that is snappy will engage users much more. In frontend controllers you often need to access several backend services to display information. These backend services calls take some time to get the data from the backend servers. Often this is done one call after the other where the call times add up.
Suppose we have a web controller that accesses three backendservices for loading a user, getting new messages for the user and getting new job offers. The code would call the three backend services and then render the page:
case class User(email:String)
case class Message(m:String)
case class JobOffer(jo:String)
def userByEmail(email:String):User = User(email)
def newMessages:Seq[Message] = Seq(
Message("Hello World"),
Message("Bye!"))
def newOffers:Seq[JobOffer] = Seq(JobOffer("Janitor")
// On our frontend we would use these services to
// display a user home page.
val user = userByEmail("stephan@ubercto.de") // takes 100ms
val messages = newMessages // takes 200ms
val offers = newOffers // takes 100ms
// render("Startpage", user, messages, newOffers)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
case class User(email:String)
case class Message(m:String)
case class JobOffer(jo:String)
def userByEmail(email:String):User = User(email)
def newMessages:Seq[Message] = Seq(
Message("Hello World"),
Message("Bye!"))
def newOffers:Seq[JobOffer] = Seq(JobOffer("Janitor")
// On our frontend we would use these services to
// display a user home page.
val user = userByEmail("stephan@ubercto.de") // takes 100ms
val messages = newMessages // takes 200ms
val offers = newOffers // takes 100ms
// render("Startpage", user, messages, newOffers)
All three calls need to be executed to render the HTML for the page. With the timings of the three calls, the rendering will take at least 400ms. It would be nice to execute all three in parallel to speed up page rendering. To achieve this we can modify our service calls to return Futures.
import scala.concurrent._
import scala.concurrent.ExecutionContext.Implicits.global
def userByEmail(email:String):Future[User] = Future {
User(email)
}
def newMessages:Future[Seq[Message]] = Future {
Seq(Message("Hello World"), Message("Bye!"))
}
def newOffers:Future[Seq[JobOffer]] = Future {
Seq(JobOffer("Janitor"))
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
import scala.concurrent._
import scala.concurrent.ExecutionContext.Implicits.global
def userByEmail(email:String):Future[User] = Future {
User(email)
}
def newMessages:Future[Seq[Message]] = Future {
Seq(Message("Hello World"), Message("Bye!"))
}
def newOffers:Future[Seq[JobOffer]] = Future {
Seq(JobOffer("Janitor"))
}
Futures in Scala are executed in a different thread from a thread pool. Futures are boxes or wrappers around values that wrap the parallel execution of a calculation and the future value of that calculation. After the Future is finished the value is available. With Futures in place the code will take only 200ms to execute instead of 400ms as in the first version. This leads to faster response times from our website.
How to work with the value inside a Future? Suppose we want the email address of the User. We could wait until the execution of the service call is finished and get the value. This would diminish the value of our parallel execution. Better we can work with the value in the future!
val user = userByEmail("stephan@ubercto.de")
// Future[String]
val email = user.map { _.email }
// non concurrent: user.email
1
2
3
4
5
6
7
val user = userByEmail("stephan@ubercto.de")
// Future[String]
val email = user.map { _.email }
// non concurrent: user.email
This way the mapping function is called when the future is completed but our code still runs in parallel.
Getting the Value From a Future
When is a Future executed and the value rendered in a web framework? When we think of the Future as a box, at one point someone needs to open the box to get the value. We can open the box and get the value of all combined futures with Await. Await also takes a timeout value:
import scala.concurrent.duration._
// Future[String]
val emailF = userByEmail("stephan@ubercto.de")
.map { _.email }
// String
val email = Await.result(emailF, 1.seconds)
// render(email)
1
2
3
4
5
6
7
8
9
10
import scala.concurrent.duration._
// Future[String]
val emailF = userByEmail("stephan@ubercto.de")
.map { _.email }
// String
val email = Await.result(emailF, 1.seconds)
// render(email)
Await waits for the Future to return and in this case with a maximum execution time of 1 second. After this we can hand the email to our web templating for rendering the template. We want to open the box as late as possible as opening each box is blocking a thread which we want to prevent.
WebFramework that Supports Futures
So why open the box at all? Better yet to use a web framework that can handle asynchronicity natively like Play Framework.
// returns Future[String]
val emailF = userByEmail("stephan@ubercto.de")
.map { _.email }
// returns Future[Result] to the request handler
emailF.map { email => Ok(dashboard.render(email) }
1
2
3
4
5
6
7
// returns Future[String]
val emailF = userByEmail("stephan@ubercto.de")
.map { _.email }
// returns Future[Result] to the request handler
emailF.map { email => Ok(dashboard.render(email) }
Play can directly work with the Future and return the result to the browser as soon as the Future is completed, while our own code finishes and frees the request thread.
Combining Futures into new Futures
We do not want to use Await for every Future or hand every Future to the async web framework. We want to combine Futures into one Future and work with this combined Future. How do you combine the different futures from the backend calls into one result? We can use map as above and then nest service calls.
// Returns
// Future[Future[Future[(User, Seq[Message], Seq[JobOffer])]]]
userByEmail("stephan@ubercto.de").map { user =>
newMessages.map { messages => {
newOffers.map { offers => (user,messages,offers) }
}
}}
1
2
3
4
5
6
7
8
// Returns
// Future[Future[Future[(User, Seq[Message], Seq[JobOffer])]]]
userByEmail("stephan@ubercto.de").map { user =>
newMessages.map { messages => {
newOffers.map { offers => (user,messages,offers) }
}
}}
Ordinary map calls do not work, as we get a deeply nested Future. So for the outer map method calls we use flatMap to flatten a Future[Future[A]] result into a Future[A]
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
userByEmail("stephan@ubercto.de").flatMap { user =>
newMessages.flatMap { messages => {
newOffers.map { offers => (user,messages,offers) }
}
}}
1
2
3
4
5
6
7
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
userByEmail("stephan@ubercto.de").flatMap { user =>
newMessages.flatMap { messages => {
newOffers.map { offers => (user,messages,offers) }
}
}}
With many service calls this becomes unreadable though.
Serial Execution
Scala has a shortcut for nested flatMap calls in the form of for comprehensions. Scala for comprehensions – which are syntactic sugar for flatMap and filter – make the code more readable. The yield block is executed when all Futures have returned.
Example: Serial execution
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
// ** NOT EXECUTED IN PARALLEL **
val result = for (
user <- userByEmail("stephan@ubercto.de"); // User
messages <- newMessages; // Seq[Messages]
offers <- newOffers // Seq[JobOffer]
) yield {
// do something here
(user, messages, offers)
}
1
2
3
4
5
6
7
8
9
10
11
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
// ** NOT EXECUTED IN PARALLEL **
val result = for (
user <- userByEmail("stephan@ubercto.de"); // User
messages <- newMessages; // Seq[Messages]
offers <- newOffers // Seq[JobOffer]
) yield {
// do something here
(user, messages, offers)
}
The for comprehension is desugared into a chain of flatMaps (see above) and the methods are called after each other and therefor the futures are created after each other. This also means the futures are not executed in parallel.
Sometimes we want to execute Futures in serial one after the other but stop after the first failure. Michael Pollmeier has an example:
serialiseFutures(List(10, 20)) { i ?
Future {
Thread.sleep(i)
i * 2
}
}
1
2
3
4
5
6
7
serialiseFutures(List(10, 20)) { i ?
Future {
Thread.sleep(i)
i * 2
}
}
which can be implemented by:
def serialiseFutures[A, B](l: Iterable[A])(fn: A => Future[B])
(implicit ec: ExecutionContext): Future[List[B]] =
l.foldLeft(Future(List.empty[B])) {
(previousFuture, next) =>
for {
previousResults <- previousFuture
next <- fn(next)
} yield previousResults :+ next
}
1
2
3
4
5
6
7
8
9
10
def serialiseFutures[A, B](l: Iterable[A])(fn: A => Future[B])
(implicit ec: ExecutionContext): Future[List[B]] =
l.foldLeft(Future(List.empty[B])) {
(previousFuture, next) =>
for {
previousResults <- previousFuture
next <- fn(next)
} yield previousResults :+ next
}
Parallel Execution
For parallel execution of the futures we need to create them before the for comprehension.
Example: Parallel execution
// Futures are created here
val userF = userByEmail("stephan@ubercto.de");
val messagesF = newMessages
val offersF = newOffers
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
// ** Executed in parallel **
for (
user <- userF;
messages <- messagesF;
offers <- offersF
) yield {
(user, messages, offers)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// Futures are created here
val userF = userByEmail("stephan@ubercto.de");
val messagesF = newMessages
val offersF = newOffers
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
// ** Executed in parallel **
for (
user <- userF;
messages <- messagesF;
offers <- offersF
) yield {
(user, messages, offers)
}
Sitenote: If you’re more into FP you can use Applicatives to combine Future results into a tuple.
Working with dependent service calls
What happens if two service calls depend on each other? For example the messages call depends on the user call. With for comprehensions this is easy to solve as each line can depend on former lines.
// Working with dependend calls
def newMessages(user:User):Future[Seq[Message]] = Future {
Seq(Message("Hello " + user.email), Message("Bye!"))
}
def userByEmail(email:String):Future[User] = Future {
User(email)
}
def newOffers:Future[Seq[JobOffer]] = Future {
Seq(JobOffer("Janitor"))
}
// Future is created here, runs in parallel
val offersF = newOffers
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
for (
user <- userByEmail("stephan@ubercto.de");
messages <- newMessages(user); // userByEmail and messages
// run in serial
offers <- offersF // This runs in parallel
) yield {
(user, messages, offers)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
// Working with dependend calls
def newMessages(user:User):Future[Seq[Message]] = Future {
Seq(Message("Hello " + user.email), Message("Bye!"))
}
def userByEmail(email:String):Future[User] = Future {
User(email)
}
def newOffers:Future[Seq[JobOffer]] = Future {
Seq(JobOffer("Janitor"))
}
// Future is created here, runs in parallel
val offersF = newOffers
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
for (
user <- userByEmail("stephan@ubercto.de");
messages <- newMessages(user); // userByEmail and messages
// run in serial
offers <- offersF // This runs in parallel
) yield {
(user, messages, offers)
}
Now userByEmail and newMessages run in serial, as the second depends on the first, while the call to newOffers runs in parallel, as we create the Future before the for comprehension and therefor it starts to run before.
The nice thing about Futures is how composable they are. For cleaner code we can create a method userWithMessages and reuse this in our final for comprehension. This way the code is easier to understand, parts are reusable and serial and parallel execution can easier be seen:
// Runs here
val offersF = newOffers
// Needs to run in serial as newMessages depends on userByEmail
def userWithMessages(email:String):Future[(User,Seq[Message])] = for (
user <- userByEmail(email);
messages <- newMessages(user)
) yield (user,messages)
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
for (
(user,messages) <- userWithMessages("stephan@ubercto.de");
offers <- offersF
) yield {
(user, messages, offers)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Runs here
val offersF = newOffers
// Needs to run in serial as newMessages depends on userByEmail
def userWithMessages(email:String):Future[(User,Seq[Message])] = for (
user <- userByEmail(email);
messages <- newMessages(user)
) yield (user,messages)
// Returns Future[(User, Seq[Message], Seq[JobOffer])]
for (
(user,messages) <- userWithMessages("stephan@ubercto.de");
offers <- offersF
) yield {
(user, messages, offers)
}
Using the method call userWithMessages in the for comprehension block works here, because it is the first and only method call. With several calls like above use
val userWithMessages = userWithMessages(...)
1
2
val userWithMessages = userWithMessages(...)
to execute in parallel before the for comprehension block.
Turning a Sequence of Futures into a Future of Sequence
If we want to get several users and our API does not support usersByEmail(Seq[String]) to get many users with one call we need to call the service for all users.
val users = Seq(
"stephan@ubercto.de",
"stefanie@eventsofa.de")
// Seq[Future]
users.map(userByEmail)
1
2
3
4
5
6
7
val users = Seq(
"stephan@ubercto.de",
"stefanie@eventsofa.de")
// Seq[Future]
users.map(userByEmail)
Here the usage of futures has major benefits as it dramatically speeds up execution. Suppose we have 10 calls with 100ms each then the serial version will take 10x100ms (1 second) whereas a parallel version from above will take 100ms.
How do we compose the result of Seq[Future[A]] with other futures? We need to turn this Sequence of Futures into a Future of Sequence. There is a method for this:
// Turns Seq[Future[User]] into Future[Seq[User]]
Future.sequence(users)
1
2
3
// Turns Seq[Future[User]] into Future[Seq[User]]
Future.sequence(users)
The future returns when all futures have returned.
Using Future.traverse
Another way is to directly use traverse
// returns Seq[Future[User]
users.map(userByEmail)
// returns Future[Seq[User]]
Future.traverse(users)(userByEmail) /
1
2
3
4
5
6
// returns Seq[Future[User]
users.map(userByEmail)
// returns Future[Seq[User]]
Future.traverse(users)(userByEmail) /
Composing Futures that contain Options
Often service calls or database calls return Option[A] for the possibility that no return value exists e.g. in the database. Suppose or User Server returns Future[Option[User]] and we want to work with the user. Sadly this does not work:
def userByEmail(email:String):Future[Option[User]] = Future {
Some(User(email))
}
// Does not work
for (
// Returns Option[User] not User
userO <- userByEmail("stephan@ubercto.de");
user <- userO; // We want the user out of the
// option but this does not work
) yield {
(user)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
def userByEmail(email:String):Future[Option[User]] = Future {
Some(User(email))
}
// Does not work
for (
// Returns Option[User] not User
userO <- userByEmail("stephan@ubercto.de");
user <- userO; // We want the user out of the
// option but this does not work
) yield {
(user)
}
This does not work as ‘for’ is syntactic sugar for flatMap, meaning the results are flattened, e.g. List[List[A]] is flattened into List[A]. But how to flat Future[Option[A]]? So composing Futures that return Options is a little more difficult. It also doesn’t work because we wrap a container (Option) into another container (Future) but want to work on the inner value with map and flatmap.
Using a FutureOption Class
One solution for keeping the Option is to write a FutureOption that combines a Future and an Option into one class. On example can be found on the edofic Blog:
case class FutureO[+A](future: Future[Option[A]]) extends AnyVal {
def flatMap[B](f: A => FutureO[B])
(implicit ec: ExecutionContext): FutureO[B] = {
FutureO {
future.flatMap { optA =>
optA.map { a =>
f(a).future
} getOrElse Future.successful(None)
}
}
}
def map[B](f: A => B)
(implicit ec: ExecutionContext): FutureO[B] = {
FutureO(future.map(_ map f))
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
case class FutureO[+A](future: Future[Option[A]]) extends AnyVal {
def flatMap[B](f: A => FutureO[B])
(implicit ec: ExecutionContext): FutureO[B] = {
FutureO {
future.flatMap { optA =>
optA.map { a =>
f(a).future
} getOrElse Future.successful(None)
}
}
}
def map[B](f: A => B)
(implicit ec: ExecutionContext): FutureO[B] = {
FutureO(future.map(_ map f))
}
}
In our example from above it could be used like this:
val userFO = for (
user <- FutureO(userByEmail("stephan@ubercto.de"));
// depend on user here
) yield {
// do something with user here
(user)
})
// Future[Option[User]]
val user = userFO.future
1
2
3
4
5
6
7
8
9
10
11
val userFO = for (
user <- FutureO(userByEmail("stephan@ubercto.de"));
// depend on user here
) yield {
// do something with user here
(user)
})
// Future[Option[User]]
val user = userFO.future
Using OptionT
As this problem often arises in functional code working with containers it has been solved before with transformers. These allow stacking of containers and transform the effects of two containers (Option having the optional effect and Future the future effect) into a new container that combines these effects. Just the thing we need.
Luckily Scalaz has a generic transformer for Option called OptionT that can combine the effect of Option with another container. Combining also means that our flatMap and map methods work with the innermost value.
import scalaz.OptionT._
// With Scalaz
// Returns OptionT[Future,User]
val userOt = for (
user <- optionT(userByEmail("stephan@ubercto.de"))
// depend on user here
) yield {
// do something with user here
(user)
}
// run returns the value wrapped in the containers
// before the transformation
// Future[Option[User]]
val user = userOt.run
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
import scalaz.OptionT._
// With Scalaz
// Returns OptionT[Future,User]
val userOt = for (
user <- optionT(userByEmail("stephan@ubercto.de"))
// depend on user here
) yield {
// do something with user here
(user)
}
// run returns the value wrapped in the containers
// before the transformation
// Future[Option[User]]
val user = userOt.run
This way we can compose calls that return Future[Option[A]].
Combining Option[A] with Seq[A]
Combining this with our messages service call that returns Seq[Message] gets a little more complex, but can be done.
import scalaz.OptionT._
// Returns OptionT[Future,(User, Seq[Message])]
val resultOt = for (
user <- optionT(userByEmail("stephan@ubercto.de"));
messages <- newMessages.liftM
// Can also be written as
// messages <- optionT(newMessages.map(_.some))
) yield {
(user,messages)
}
// Future[Option[(User, Seq[Message])]
val result = resultOt.run
1
2
3
4
5
6
7
8
9
10
11
12
13
import scalaz.OptionT._
// Returns OptionT[Future,(User, Seq[Message])]
val resultOt = for (
user <- optionT(userByEmail("stephan@ubercto.de"));
messages <- newMessages.liftM
// Can also be written as
// messages <- optionT(newMessages.map(_.some))
) yield {
(user,messages)
}
// Future[Option[(User, Seq[Message])]
val result = resultOt.run
The interesting line is messages <- newMessages.liftM where newMessages returns Future[Seq[Message]]. To get this working with Future[Option[A]] we can either transform this by hand or use liftM. LiftM automatically “lifts” the value into the right containers, OptionT[Future, Seq[Message]] in this case.
Combining Iterating over Lists with Futures
Sometimes you want to work with futures and iterate over Future[List[A]]. This can be achieved with nested for comprehensions:
def newMessages:Future[Seq[Message]] = Future {
Seq(Message("Hello World"), Message("Bye!"))
}
for (
user <- userByEmail("stephan@ubercto.de");
messages <- newMessages
) yield for (
message <- messages
) yield {
// Do somethin here
// Returns Future[Seq[(String, Message)]]
(user.email, message)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
def newMessages:Future[Seq[Message]] = Future {
Seq(Message("Hello World"), Message("Bye!"))
}
for (
user <- userByEmail("stephan@ubercto.de");
messages <- newMessages
) yield for (
message <- messages
) yield {
// Do somethin here
// Returns Future[Seq[(String, Message)]]
(user.email, message)
}
Error Handling
For Futures and Futures with Options all the error handling strategies exist that I wrote about in “Some thoughts on Error Handling”.
But there are more. For combining Try with Future one can convert the Try to a Future. It makes more sense to flatten Future[Try[]] than flatten Future[Option[]]. Try and Future have similar semantics – Success and Failure – whereas Future and Option differ in their semantics.
def registerUser(u:User):Try[User] = ...
val result = for{
u <- userByEmail("stephan@ubercto.de");
registered <- tryToFuture(registerUser(u))
} yield registered
result.map { /* Success Block */ } recover { /* Failure Block */ }
def tryToFuture[T](t:Try[T]):Future[T] = {
t match{
case Success(s) => Future.successful(s)
case Failure(ex) => Future.failed(ex)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
def registerUser(u:User):Try[User] = ...
val result = for{
u <- userByEmail("stephan@ubercto.de");
registered <- tryToFuture(registerUser(u))
} yield registered
result.map { /* Success Block */ } recover { /* Failure Block */ }
def tryToFuture[T](t:Try[T]):Future[T] = {
t match{
case Success(s) => Future.successful(s)
case Failure(ex) => Future.failed(ex)
}
}
Beside this Future in Scala has many ways to handle error conditions with fallbackTo, recover and recoverWith which to me are the preferred ways instead of failure callbacks which also exist.
Futures can make your frontend code faster and your customers happier. I hope this small guide helped you understanding Futures and how to handle them.
If you have ideas about handling Futures or general feedback, reply to @codemonkeyism
Notes
The article uses container or box for describing Future and Option. The term that is usually used is Monad. Often this term confuses people so I have used the simpler box and container instead.
The article assumes creating Futures creates parallel running code. This is not always the case and depends on the number of available cores and the thread pool or concurrency implementation underlying the Futures. For production deployment and performance you need to read about thread pools and how to tune them to your application and hardware. Usually an application has different thread pools e.g. for short running code, long running code or blocking (e.g. IO) code.
The article assumes that code in Future is not blocking. If you use blocking code in Futures, e.g. IO with blocking database drivers, this has impact on your performance.
Stephan the codemonkey writes some code since 35 years, was CTO in some companies and currently helps startups with their technical challenges
10 Best HTML5 Development Tools For Web Develop...
The arrival of HTML5 transformed the designing and development business and completely transformed the way development was done earlier. HTML5 carried with itself a simpler and faster way technique of development and delivered developers with a lot of diverse tools and features that can be utilized to make their job a lot cooler. Its features are extremely useful and functional which let offering online content in a much improved and enriched approach. HTML5 tools not only aid designers and developers to make their website much more attractive and enhanced beholding but also augment its usability and accessibility. These tools and features let integrating astonishing animations, effects, images, video, audio, etc. to their web pages.
Seeing the benefits of HTML5, it is fairly understandable that all developers and designers are continuously on the search for some worthy tools that can aid them to make their job stress-free, faster and hassle-free! So to aid our friends out, today we are sharing with you all an assembly of 10 Best HTML5 Development Tools to protect your Time.
10 Best HTML5 Development Tools to protect your Time
Patternizer
As the name indicates, this tool lets making truly cool designs such as lines and strips etc. You can revise your design by line width, color, angle and break etc. It is seamless for generating background pictures etc.
Font Dragr
This tool marks trying fonts for appropriateness with your project actually stress-free as it takes away all the annoyance of font codes etc, but merely lets you to check website fonts by dragging and dropping them.
Adobe Typekit
This tool aids you select the appropriate font by permitting you to sight real-time showings of your page with diverse fonts so that choosing the correct font won’t be a very hard job.
Rendera
If you desire an atmosphere where you can check, discover and research about with diverse types of CSS, HTML, or JavaScript codes. It will deliver you real-time outcomes as you type, therefore creating the coding procedure speedier and cooler.
CanvasLoader Creator
This overwhelming tool permits modifying your loader to make it appear more eye-catching and striking and offering the visitors a healthier understanding even though the entity of their selection is being loaded. It does not need any programming.
Webdirections
Webdirections is a fine concourse for codes that permits them to check, search and try-out with their codes. It has got some of the humblest click choices as HTML5 Tools, CSS Tools, and design tools etc which can be effortlessly and rapidly opened by clicks.
Initializr
Initializr is accurately named so as it allows you initiate creation from scratch by providing you with an atmosphere where you can choose which portions you would like to be encompassed in the HTML site development and which not to be included.
On/Off Flipswitch HTML5/CSS3 Generator
It lets making flip-switches that allow the people on your site to turn on/off the moving picture on the page. These changes can be made using small codes that are companionable with numerous Operating systems and web browsers.
Liveweave
This one is an overwhelming site for programmers and developers etc as it permits functioning, examination and testing on HTML5, CSS3, or JavaScript codes.
HTML KickStart
This tool really gives you a jump-start for your website construction from scratch as it permits modifying typography, tables, buttons, and lists etc with a few clicks.
英文出自:Designdrizzle
10 HTML5 text editors for web developers and designers
https://smashinghub.com/10-html5-text-editors-for-web-developers-and-designers.htm
Text editors are the essential tools for web developers. In fact, it is also not uncommon for designers to know a bit of code editing as well include HTML, CSS etc. The past text editors used to be downloadable versions where we need to install. This makes them limiting as revisions are slow to come by. With HTML5 technology, we are seeing a current new batch of HTML5 text editors that are completely written for the web. The good thing is that version changes can be fast and the capabilities of these text editors can be extended via plugins or other extensions.
Below is a roundup of some of the best and free HTML5 text editors for designers and web developers.
#1: Brackets: Adobe’s opensource text editor
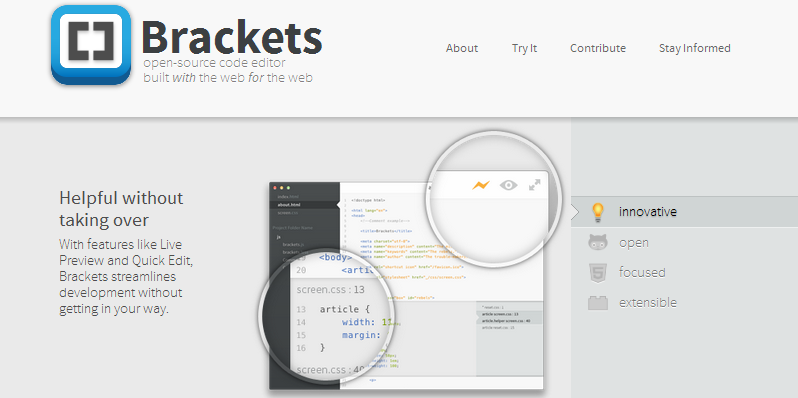
Brackets was launched last year by Adobe as an open source text editor whose capability can be extended via their bracket extensions. The entire editor was built on HTML, CSS etc which means it is very easy to work with for those who are familiar with these languages. Extensions can also be easily built based on these common web language.
#2: Popline
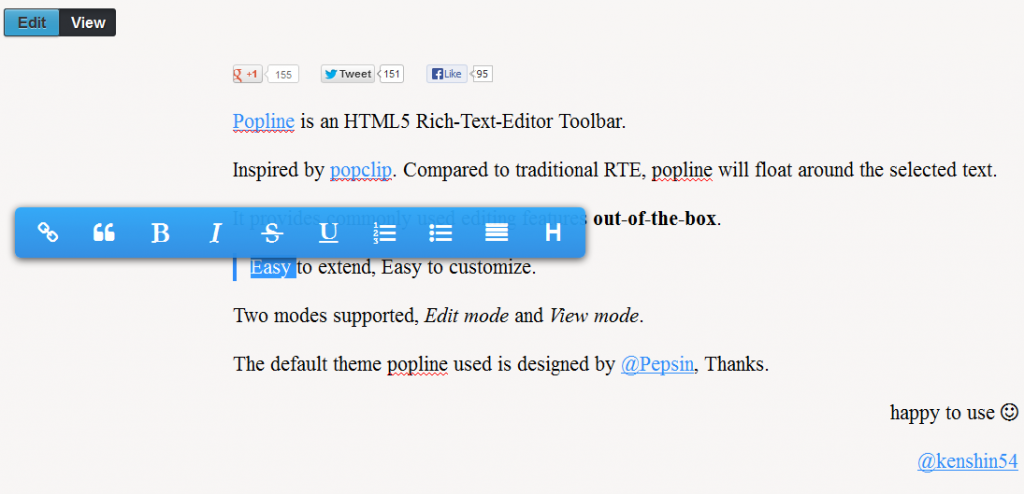
Popline is a pure HTML5 editor toolbar. It floats on the things that you want to edit so it is rather cool to use. Function wise, it does not have all the bells and whistle of traditional text editor but for designers, it is definitely sufficient.
#3: Bootstrap-wysiwyg
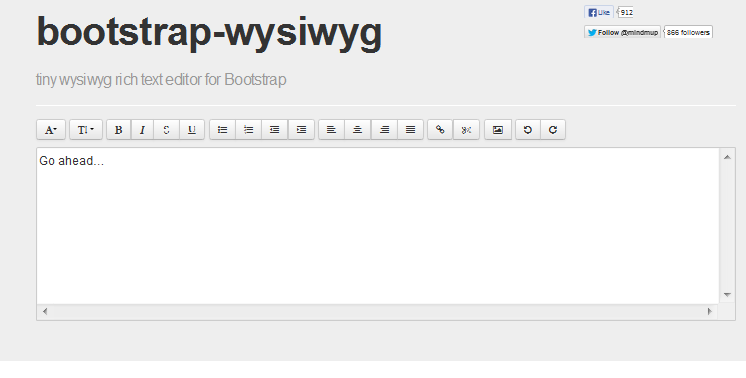
This is a simple but functional editor for bootstrap. The plugin turns any DIV into WYSIWYG rich-content editor. You can see how it looks from the screenshot above.
#4: Mercury editor
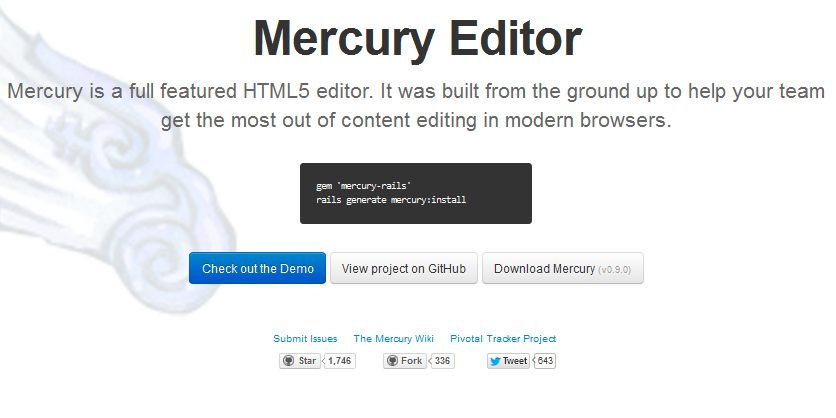
One of the most powerful HTML5 editor is the mercury editor. It has a full fledged editor with support for almost all web naive language including HTML, elements sybnax and even Javascript APIs. There is even a drag and drop function for all file uploading. Very cool.
#5: Aloha editor
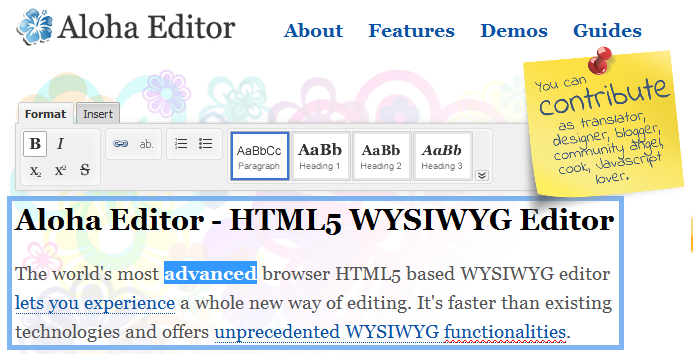
The distinct feature of aloha editor is that it can be embedded within a CMS like wordpress. This makes page editing much faster, especially if you liked to play with your page formatting and layout.
#6: jResponse – A text editor for responsive web design
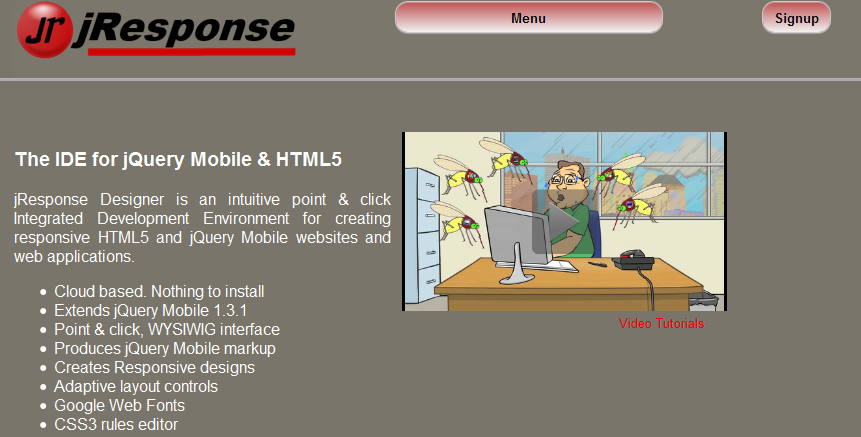
jResponse is an interesting text editor as it focuses on supporting responsive design within its framework. It is a bit rough right now as the program is still in beta but it is worth signing up and taking it for a test drive to see if it can improve our productivity when it comes to developing websites for mobile.
#7: WebMatrix3
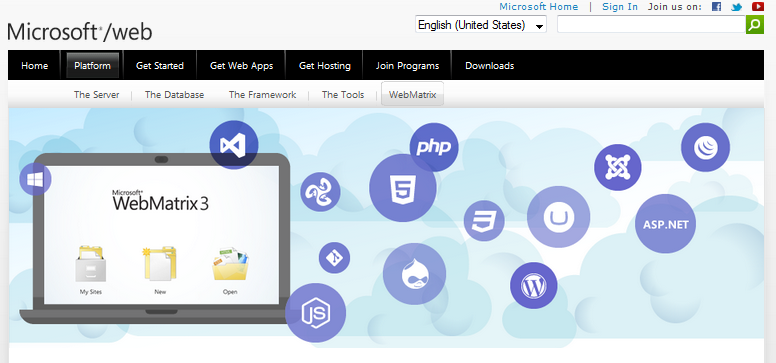
I think most will be familiar WebMatrix3 from Microsoft. It is nice HTML5 web development tool and comes with a lot of cool features such as code completion for jQuery Mobile and supporting a range of language include ASP.NET, PHP and HTML5 templates.
#8: Webstorm
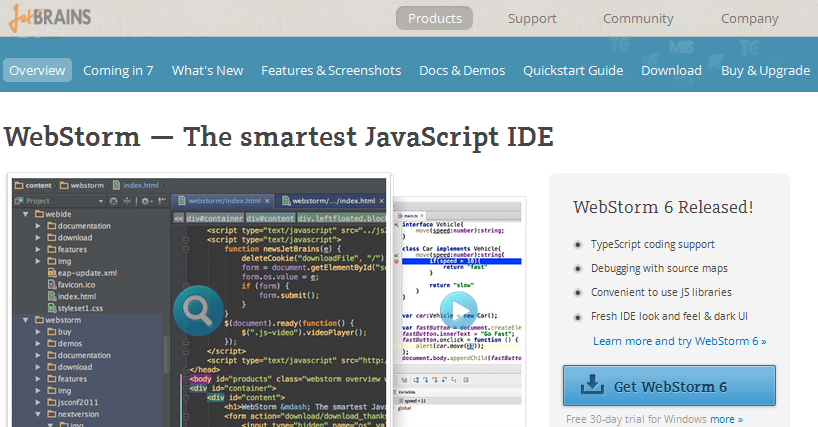
Webstorm is a cool editor that comes with loads of functions. People who have tried it will know what I am talking about. It supports a wide range of web language including HTML5. It also has a nice debugging interface that will make it faster for you to spot and repair all the bugs in your code.
#9: Raptor Editor
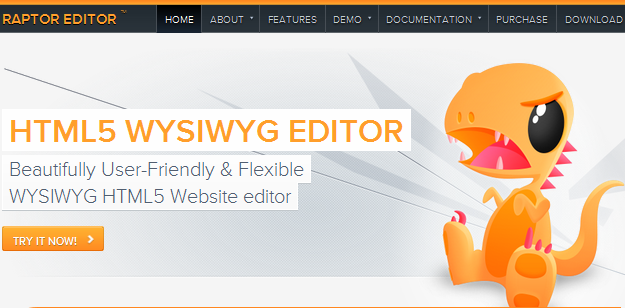
Raptor editor is one of the most beautiful html5 text editor that I have seen. Not only is it nice to look at, the editor itself is entirely modular in nature so that everything is extensible. It is literally a modern editor that is suited for both developers and designers.
#10: TopStyle 5
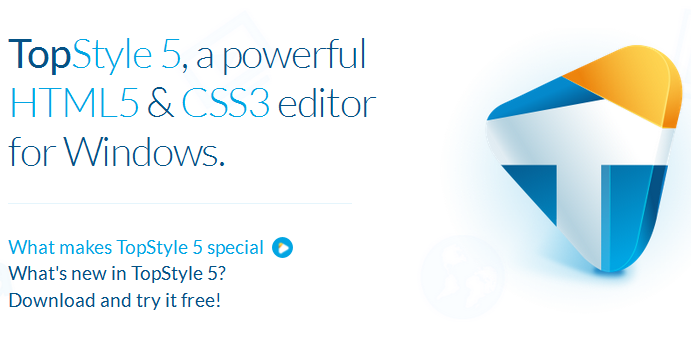
TopStyle 5 is a very modern html5 editor that has a nice preview function for you to see real time changes. This improves the productivity of your code writing quite significantly and is one of the feature I liked best.
100 Free Courses & Tutorials for Aspiring iPhone App Developers
Unless you’ve been living under a rock,you kNow that the iPhone is a big deal and it’s one of the most popular subjects of development these days. Lots of developers are creating their own iPhone apps,and with the right kNow-how,you can too. Check out our list of courses and tutorials to learn everything that’s important about developing for the iPhone.
University
Here you’ll find iPhone development courses offered by top universities.
- iPhone Application Programming: Learn about programming for the iPhone from Stanford on iTunes. [Stanford]
- Introduction to iPhone Application Development: Use this course’s posted slides to get a crash course in iPhone application development. [MIT]
Apple Resources
You can learn about iPhone development straight from the source with these Apple documents.
- Getting Started with iPhone: Here you’ll find a general introduction to iPhone development. [Apple]
- Object-Oriented Programming with Objective-C: This document offers an excellent guide for object oriented programming. [Apple]
- Networking & Internet Coding How-Tos: In this resource,you will find lots of great advice for networking and Internet development on the iPhone. [Apple]
- Getting Started with Audio & Video: Use this document to get started with audio and video features in iPhone applications. [Apple]
- Your First iPhone Application: This introductory tutorial offers a step by step description of getting started with an iPhone application. [Apple]
- Getting Started with Performance: This guide offers an introduction to improving the performance on iPhone apps. [Apple]
- iPhone Application Programming Guide: Get an introduction to the iPhone OS and development process. [Apple]
- iPhone OS Technology Overview: Learn about the iPhone OS and its technologies from this guide. [Apple]
- Getting Started with Data Management: Here you’ll find a reference that will help you with data management. [Apple]
- Security Overview: Get an understanding of the security concepts on the iPhone from this resource. [Apple]
- Performance Overview: Get a look at the factors that determine performance through this guide. [Apple]
- Resource Programming Guide: Check out this resource to learn how to work with nib and bundle resources.
- Getting Started with User Experience: This document offers an introduction to constructing iPhone application user interfaces. [Apple]
- iPhone Human Interface Guidelines: Follow these guidelines to make sure your iPhone app has a good human interface. [Apple]
- iPhone Development Guide: Use this development guide to get an introduction to creating web apps on the iPhone. [Apple]
- Data Formatting Programming Guide for Cocoa: This guide will teach you how to use Cocoa formatters for data. [Apple]
- Getting Started with Tools: You will find a guided introduction to the Xcode toolset from this document. [Apple]
- Data Management Coding How-tos: Get answers to common data management coding questions. [Apple]
- Introduction to Cocoa Application Tutorial: You’ll need at least a base level understanding of Cocoa for iPhone development,which you can check out in this tutorial. [Apple]
- Core Animation Programming Guide: Follow this guide to get the main components and services of Core Animation. [Apple]
- Coding Guidelines for Cocoa: In this guide,you’ll learn about naming guidelines for the Cocoa API as well as design advice. [Apple]
- Getting Started with Graphics and Animation: Follow this guide for an introduction to 2D and 3D graphics and animation. [Apple]
- Learning Objective-C: A Primer: Check out this document once you’ve worked through object oriented programming and Cocoa. [Apple]
- Cocoa Fundamentals Guide: You’ll learn about the basic concepts,terminology,and more in Cocoa from this guide. [Apple]
- Graphics and Animation Coding How-Tos: In this resource,you’ll find lots of great tips and advice for graphics and animation on the iPhone. [Apple]
Getting Started
Get an introduction to iPhone development through these tutorials.
- iPhone App Development-Where to Start: This tutorial will teach you how to get started in iPhone app development. [The Daleisphere]
- Bootstrap: Learn a few pointers for iPhone development from this resource. [furbo]
- Learn How to Develop for the iPhone: This tutorial will show you how to build an alternate page and style sheet for the iPhone. [NETTUTS]
- iPhone Application Development,Step By Step: In this tutorial,you will find a step by step guide to creating a simple iPhone game. [Open Laszlo]
- First iPhone Application: Get a brief introduction to creating your first iPhone application. [iPhone SDK Articles]
- iPhone Dev: Check out this PDF to get a tutorial for iPhone development. [Lucas Newman]
- iPhone App Development for Web Hackers: Use this tutorial to learn about geo-location features and beginner development tips. [How to Iphone Application]
- How to Write an iPhone App: This tutorial gives you a basic look at what it takes to write an iPhone application. [Webmonkey]
- iPhone App Development for Web Hackers: In this article,you’ll learn about web hacking development for the iPhone. [Dominiek]
- Writing Your First iPhone Application: Bill Dudney will walk you through all of the tools and pieces of kNowledge you’ll need to write your first iPhone application. [The Pragmatic Bookshelf]
- Cocoa Touch Tutorial: iPhone Application Example: This tutorial will show you how to make a very basic Cocoa Touch application with Interface Builder. [Cocoa Is My Girlfriend]
- Building an iPhone app in a day: Check out this tutorial to see how you can build a useful app quickly. [The Bakery]
- Seven Things All iPhone Apps Need: Check out this list to see what’s essential when creating an iPhone app. [APCmag]
- Put Your Content in My Pocket: Learn how to use the iPhone web browser to your advantage from this article. [A List Apart]
- iPhone Training Course: Become a master at writing iPhone applications through this course. [Rose India]
- So you’re going to write an iPhone app…: Learn about code reuse,memory,and more from this tutorial. [furbo]
- Learn How to Develop for the iPhone: Check out this tutorial to see how to build an alternative page and style sheet for the iPhone. [Net Tuts]
- Developing for the iPhone: This resource will show you how to develop ASP.NET applications for the iPhone. [Dot Net Slackers]
- Getting Started with iPhone Development: Ed Burnette offers a basic introduction to iPhone development. [ZDnet]
Tools
These tutorials will teach you how to use specific tools in order to create iPhone apps.
- Make an iPhone App Using the Envato API: Make your own iPhone app with the Envato API with the help of this tutorial. [Net Tuts]
- Developing iPhone Applications using Ruby on Rails and Eclipse: Learn how to detect mobile Safari from a Ruby on Rails application through this tutorial. [IBM]
- 14 Essential Xcode Tips,Tricks and Resources for iPhone Devs: Learn how to make sense of xcode with this helpful resource. [Mobile Orchard]
- Develop iPhone Web Applications with Eclipse: This tutorial will help you learn how to create iPhone applications with Aptana’s iPhone development plug-in. [IMB]
- Build an iPhone Webapp in Minutes with Ruby,Sinatra,and iUI: You can learn how to quickly put together an iPhone app with these tools. [Mobile Orchard]
- iPhone Development with PHP and XML: In this tutorial,you’ll get a look at developing custom applications for the iPhone. [IBM]
Details
These tutorials cover all of the important details in iPhone app development.
- Avoiding iPhone App Rejection from Apple: This tutorial holds the secrets to making sure your iPhone app makes the cut. [Mobile Orchard]
- Landscape Tab Bar Application for the iPhone: Follow this tutorial to learn about making the tab bar application support landscape orientation. [Cocoa Is My Girlfriend]
- iPhone Programming Tutorial-Using openURL to Send Email from Your App: This tutorial explains how you can send email through applications,and even pre-fill fields. [iCode]
- Multi Touch Tutorial: This tutorial will show you how you can respond to a tap event. [iPhone SDK Articles]
- Create a Navigation-Based Application: This tutorial will teach you how to create and run a navigation-based application from XCode.
- Advanced iPhone Development: Go beyond the basics with this iPhone development tutorial. [Dot Net Slackers]
- Here’s a Quick Way to Deal with Dates in Objective C: Get information on dealing with date fetching through this tutorial. [Howtomakeiphoneapps]
- Navigation Controller + UIToolbar: Through this tutorial,you can learn how to add a UIToolbar to an app. [iPhone SDK Articles]
- iPhone Asynchonous Table Image: Follow this thorough article to learn about loading multiple images in your iPhone app in an asynchonous manner. [Markj]
- Localizing iPhone Apps-Internationalization: You can use resource files to display text in a user’s language-learn how in this tutorial. [iPhone SDK Articles]
- Tutorial: JSON Over HTTP on the iPhone: With this tutorial,you’ll get a step by step how-to for JSON web services through an iPhone app. [Mobile Orchard]
- Parsing xml on the iPhone: This tutorial will show you how to parse XML using the iPhone SDK. [Craig Giles]
- Reading data from a SQLite Database: Here you’ll find a quick tutorial for reading data from a sqlite database. [dBlog]
- How to Make an Orientation-Aware Clock: Through this tutorial,you’ll learn about building a simple,orientation-aware clock. [The Apple Blog]
- Finding iPhone Memory Leaks: Carefully find iPhone memory leaks by using this tutorial. [Mobile Orchard]
- Localizing iPhone Apps: MAke sure that your iPhone app is properly formatted according to a user’s native country or region with the help of this tutorial. [iPhone SDK Articles]
- OpenAL Audio Programming on iPhone: Here you’ll get code snippets,learning,and more. [Gehaktes]
- 9 iPhone Memory Management Links and Resources: Here you’ll find a variety of iPhone memory management resources that can help you get things under control. [Mobile Orchard]
- Parsing XML Files: Get an understanding of how you can parse XML files with this tutorial. [iPhone SDK Articles]
User Interface
These tutorials are all about the user interface and interaction.
- UITableView-Drill down table view tutorial: Check out this tutorial to learn how to make a drill down table view. [iPhone SDK Articles]
- iPhone Coding-Learning About UIWebViews by Creating a Web Browser: In this tutorial,you’ll learn about UIWebViews through the creation of a browser. [iCode]
- Design Patterns on the iPhone: Check out David Choi’s guest lecture on user interface design for the iPhone. [New Jersey Institute of Technology]
- UITableView-Adding subviews to a cell’s content view: This tutorial will show you how to customize the UITableViewCell. [iPhone SDK Articles]
- Drill down table view with a detail view: Learn how to load a different detail view on the UITabBarController. [iPhone SDK Articles]
- Extending the iPhone’s SDK’s UIColor Class: Learn how to extend the iPhone SDK UIColor class,and get code samples from this article. [Ars Technica]
- UITableView: Learn how to make a simple index for the table view with this tutorial. [iPhone SDK Articles]
Building Tutorials
Check out these tutorials where you’ll build a specific app,and learn more about iPhone development along the way.
- Build a Simple RSS Reader for the iPhone: Get walked through the creation of an RSS reader for a simple Feed on the iPhone. [The Apple Blog]
- iPhone Gaming Framework: This article offers a look at writing code for iPhone game developers. [Craig Giles]
- Build a Simple RSS Reader for the iPhone: Follow this tutorial,and you’ll learn about building a simple iPhone RSS reader.
- iPhone Game Programming Tutorial: This multipart tutorial offers a way to learn OpenGL and Quartz for iPhone development. [iCode]
- Build your very own Web browser!: Follow this tutorial to learn about the process of building your own iPhone web browser. [dBlog]
- iPhone application development,step by step: Find out how to build the iPhone application NEWSMATCH using OpenLaszlo. [OpenLaszlo]
- Building an Advanced RSS Reader using TouchXML: Get step by step information for creating an advanced iPhone RSS reader from this tutorial. [DBlog]
- iPhone SDK Tutorial: Building an Advanced RSS Reader Using TouchXML: This tutorial will help you learn more about iPhone development by building an advanced RSS reader with TouchXML. [dBlog]
Videos
Watch these videos for a visual guide to iPhone app development.
- Basic iPhone Programming: Check out this video to get started with iPhone programming. [iPhone Dev Central]
- First Step Towards the App Store: Work towards getting your app in the app store with the help of this tutorial. [You Tube]
- Hello World: This tutorial will help you learn the basics of iPhone programming. [iPhone Dev Central]
- UITableView iPhone Programming Tutorial: Watch this video to learn how to populate a UITableView. [YouTube]
- iPhone App Tutorial 1: Check out this video to quickly learn about Interface Builder. [YouTube]
- iPhone IB-Your First App: Watch this tutorial to learn how to use the Interface Builder. [iPhone Dev Central]
- Understanding Source Code: Learn how to get started with development on the iPhone through this video tutorial. [YouTube]
- How to Make an iPhone App: Create an iPhone app using Jiggy and this tutorial. [YouTube]
- iPhone Development with Dashcode: Find out how to develop iPhone applications with Dashcode through this tutorial. [YouTube]
Development Resources
These resources are not courses or tutorials,but they are incredibly valuable resources for beginner iPhone app developers.
- iPhone Open Application Development: This book will teach you how to create software for the iPhone environment. [Safari Books Online]
- iPhone GUI PSD File: Use this set to get a comprehensive,editable library of iPhone UI assets. [Teehanlax]
- 31 iPhone Applications with Source Code: Teach yourself how to create iPhone apps by taking a look at the code in these. [Mobile Orchard]
- iPhoney: Using iPhoney,you’ll be able to see how your creation will look on the iPhone. [Market Circle]
- 35 Free iPhone Icon Sets: Check out this resource to find a great variety of iPhone icons.
From: http://www.bestuniversities.com/blog/2009/100-free-courses-tutorials-for-aspiring-iphone-app-developers/
8 Python Frameworks For Web Developers
Python has become immensely popular in the modern IT world. The language is most popular for its efficiency. It is also known as the best beginner’s learning language. The prime reason why Python has become so popular is because of the simplistic code. Python has powerful constructs for high speed development. The standard library of python n is huge. Today we have listed top eight Python frameworks that you can use in your web development project.
1. Django:
Django has number of individual benefits. The tool helps in reducing the size of the code. It also enables fast and easy web development process. High-end developers always prefer Django over all other frameworks. The tool follows DRY (Don’t Repeat Yourself) principal. You can use Django to reuse the code for quicker development.
2. Flask:
Flask is microframework that can extend its simple core. A new programmer might find it very difficult to use it as it lacks several important features. The tool allows extensions that make it comparatively easier to add required functionality to your Java code. Some of the features of Flask are perfect for development like unit testing. Flask allows secure cookies for client applications.
3. CherryPy:
CherryPy is important tool for python development. The tool has pythonic interface that lets developers integrate any module in python. The best part of CherryPy is the ability to customize each function and its native adapter. CherryPy offers most of the WSGI-enabled adapter support.
4. Pyramid:
Pyramid is known for its efficient and fast-pace development abilities. The best part of Pyramid framework is inclusion of some of the most exclusive features. The open source framework has platform independent MVC structure and minimalistic approach in development.
5. Web.py:
Web.py is the unique python framework. The framework is known for its simplistic approach and powerful development ability. You can easily write web apps using Web.py. The framework offers zero limitations and ease of user. Some programmers find that Web.py lacks certain features.
6. Grok:
Grok is based on Zope toolkit. The framework emerged as an extension of Zope to make it easier for programmers to use. Grok can offer multiple building blocks and excellent community. Grok follows DRY approach.
7. Pylons:
Pylons offers great flexibility to developers. The framework is based on an idea to combine some of the best feature offered by numerous Python frameworks. You will find all important features of different frameworks in combined form in Pylons. You can use any feature of your choice to make web development efficient.
8. TurboGears:
This is a popular conclusive framework with all the features of other Python frameworks. You can extend its capabilities to use it as full-stock solution. TurboGears is also useful for micro framework projects. You never feel like you are working on a framework. TurboGears feels like you are writing new functions. You can create read-to-extend application under a minute using TurboGears.
关于A LITTLE GUIDE ON USING FUTURES FOR WEB DEVELOPERS的介绍现已完结,谢谢您的耐心阅读,如果想了解更多关于10 Best HTML5 Development Tools For Web Develop...、10 HTML5 text editors for web developers and designers、100 Free Courses & Tutorials for Aspiring iPhone App Developers、8 Python Frameworks For Web Developers的相关知识,请在本站寻找。
本文标签: