string path=Server.MapPath("C#语言参考.doc");
FileInfo file=new FileInfo(path);
FileStream myfileStream=new FileStream(path,FileMode.Open,FileAccess.Read);
byte[] filedata=new Byte[file.Length];
myfileStream.Read(filedata,(int)(file.Length));
myfileStream.Close();
Response.Clear();
Response.ContentType="application/msword";
response.addheader("Content-disposition","attachment;filename=文件名.doc");
Response.Flush();
Response.BinaryWrite(filedata);
Response.End();
您可能感兴趣的文章:
- C#对Word文档的创建、插入表格、设置样式等操作实例
- C#设置Word文档背景的三种方法(纯色/渐变/图片背景)
- C# 向Word中设置/更改文本方向的方法(两种)
- C#添加、读取Word脚注尾注的方法
- C# WORD操作实现代码
- C#实现通过模板自动创建Word文档的方法
- C# Word 类库的深入理解
- 比较全的一个C#操作word文档示例
- C# 利用Aspose.Words.dll将 Word 转成PDF
- C#如何更改Word的语言设置
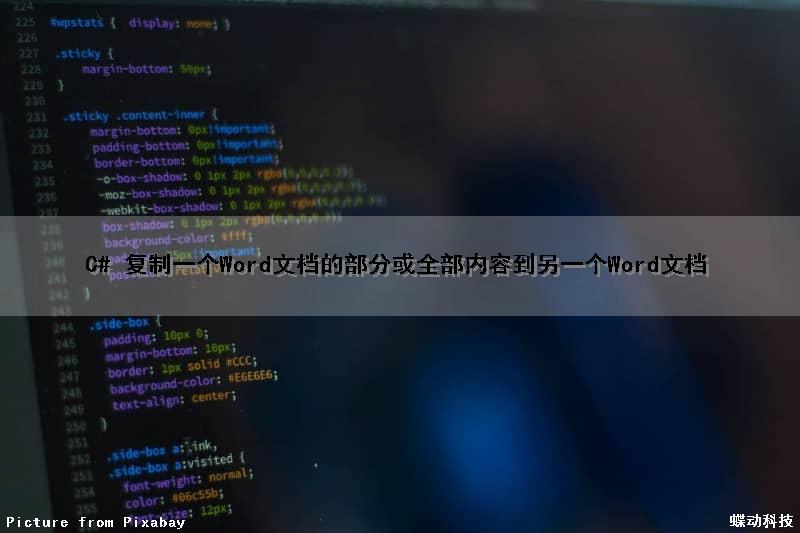
C# 复制一个Word文档的部分或全部内容到另一个Word文档
C# 复制一个Word文档的部分或全部内容到另一个Word文档
我最近喜欢折腾Office软件相关的东西,想把很多Office软件提供的功能用.NET来实现,如果后期能把它用来开发一点我自己的小应用程序那就更好了。
扯远了,回到正题。复制文档内容这个功能太常见啦,在微软Word中实现这个功能很简单,只需要复制和粘贴就行了。这篇文章的主要目的是记录如何用C#来实现复制一个Word文档的部分或全部内容到另一个word文档,废话不多说,下面开始。
第一部分:复制部分内容:
在我的这个示例中,复制部分内容指的是复制一个word文档的部分段落(包括格式、图片和超链接等)到另一个word文档。
原文档截图:

步骤参考:
第一步:新建一个word文档对象doc1并加载需要复制的word文档。
Document doc1 = new Document();
doc1.LoadFromFile("sample.docx");
第二步:新建一个word文档对象doc2。
Document doc2 = new Document();
第三步:获取被复制文档doc1的第一个section以及第一、二个段落(图片和标题)。
Section s = doc1.Sections[0];
Paragraph p1 = s.Paragraphs[0];
Paragraph p2 = s.Paragraphs[1];
第四步:给doc2添加一个section,并将doc1的第一二段的内容和格式等复制到doc2中。
Section s2 = doc2.AddSection();
Paragraph NewPara1 = (Paragraph)p1.Clone();
s2.Paragraphs.Add(NewPara1);
Paragraph NewPara2 = (Paragraph)p2.Clone();
s2.Paragraphs.Add(NewPara2);
第五步:保存并重新打开文档。
doc2.SavetoFile("copy.docx",FileFormat.Docx2010);
System.Diagnostics.Process.Start("copy.docx");
目标文档效果图:

第二部分:复制全部内容
复制全部内容指的是将除header和footer以外的其他所有内容复制到另一个文档。
步骤参考:
第一步:新建两个word document对象,并加载待复制的源word文档和目标word文档。
Document sourceDoc = new Document("sample.docx");
Document destinationDoc = new Document("target.docx");
第二步:遍历源word文档中的所有section并把它们的内容复制到目标word文档。
foreach (Section sec in sourceDoc.Sections)
{
foreach (DocumentObject obj in sec.Body.Childobjects)
{
destinationDoc.Sections[0].Body.Childobjects.Add(obj.Clone());
}
}
第三步:保存并重启目标word文档。
destinationDoc.SavetoFile("target.docx");
System.Diagnostics.Process.Start("target.docx");
在word文档之间单独复制header和footer也可以实现的,如有需要可以在下面留言。
原文档与效果图:

效果图:

结语:
文章写的比较简单,这里我还是使用了E-iceblue公司的免费Word控件,原因是它简单好用,而且我也使用的比较熟练了。接下来我还会研究它的其它功能,并用C#实现Office软件的其它功能。
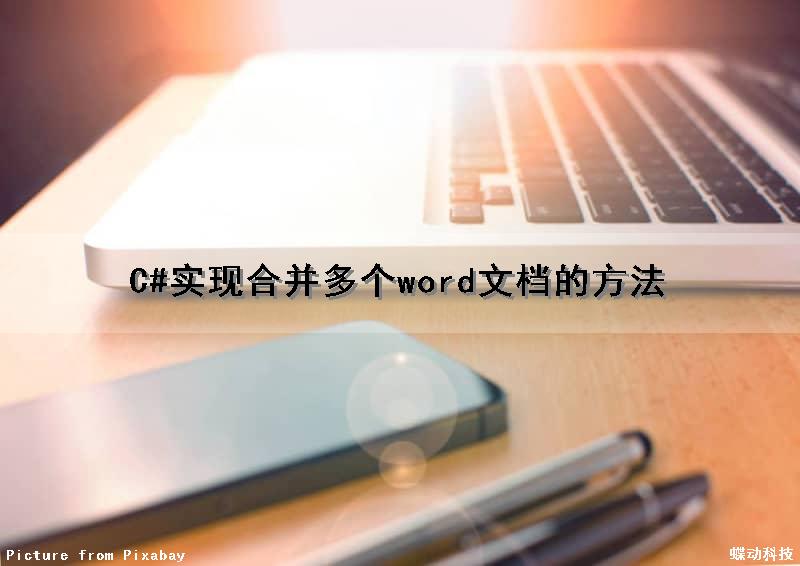
C#实现合并多个word文档的方法
本文实例讲述了C#实现合并多个word文档的方法,是非常具有实用价值的技巧。分享给大家供大家参考。
具体实现方法如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Microsoft.Office.Interop.Word;
using System.Reflection;
using System.IO;
using System.Diagnostics;
namespace driverexam.WordReport
{
public class WordDocumentMerger
{
private ApplicationClass objApp = null;
private Document objDocLast = null;
private Document objDocBeforeLast = null;
public WordDocumentMerger()
{
objApp = new ApplicationClass();
}
#region 打开文件
private void Open(string tempDoc)
{
object objTempDoc = tempDoc;
object objMissing = System.Reflection.Missing.Value;
objDocLast = objApp.Documents.Open(
ref objTempDoc,//FileName
ref objMissing,//ConfirmVersions
ref objMissing,//ReadOnly
ref objMissing,//AddToRecentFiles
ref objMissing,//PasswordDocument
ref objMissing,//PasswordTemplate
ref objMissing,//Revert
ref objMissing,//WritePasswordDocument
ref objMissing,//WritePasswordTemplate
ref objMissing,//Format
ref objMissing,//Enconding
ref objMissing,//Visible
ref objMissing,//OpenAndRepair
ref objMissing,//DocumentDirection
ref objMissing,//NoEncodingDialog
ref objMissing //XMLTransform
);
objDocLast.Activate();
}
#endregion
#region 保存文件到输出模板
private void SaveAs(string outDoc)
{
object objMissing = System.Reflection.Missing.Value;
object objOutDoc = outDoc;
objDocLast.SaveAs(
ref objOutDoc,//FileFormat
ref objMissing,//LockComments
ref objMissing,//PassWord
ref objMissing,//WritePassword
ref objMissing,//ReadOnlyRecommended
ref objMissing,//EmbedTrueTypeFonts
ref objMissing,//SaveNativePictureFormat
ref objMissing,//SaveFormsData
ref objMissing,//SaveAsAOCELetter,ref objMissing,//Encoding
ref objMissing,//InsertLineBreaks
ref objMissing,//AllowSubstitutions
ref objMissing,//LineEnding
ref objMissing //AddBiDiMarks
);
}
#endregion
#region 循环合并多个文件(复制合并重复的文件)
/// <summary>
/// 循环合并多个文件(复制合并重复的文件)
/// </summary>
/// <param name="tempDoc">模板文件</param>
/// <param name="arrcopies">需要合并的文件</param>
/// <param name="outDoc">合并后的输出文件</param>
public void copyMerge(string tempDoc,string[] arrcopies,string outDoc)
{
object objMissing = Missing.Value;
object objFalse = false;
object objTarget = WdMergeTarget.wdMergeTargetSelected;
object objUseFormatFrom = WdUseFormattingFrom.wdFormattingFromSelected;
try
{
//打开模板文件
Open(tempDoc);
foreach (string strcopy in arrcopies)
{
objDocLast.Merge(
strcopy,//FileName
ref objTarget,//MergeTarget
ref objMissing,//DetectFormatChanges
ref objUseFormatFrom,//UseFormattingFrom
ref objMissing //AddToRecentFiles
);
objDocBeforeLast = objDocLast;
objDocLast = objApp.ActiveDocument;
if (objDocBeforeLast != null)
{
objDocBeforeLast.Close(
ref objFalse,//SaveChanges
ref objMissing,//OriginalFormat
ref objMissing //RouteDocument
);
}
}
//保存到输出文件
SaveAs(outDoc);
foreach (Document objDocument in objApp.Documents)
{
objDocument.Close(
ref objFalse,//SaveChanges
ref objMissing,//OriginalFormat
ref objMissing //RouteDocument
);
}
}
finally
{
objApp.Quit(
ref objMissing,//SaveChanges
ref objMissing,//OriginalFormat
ref objMissing //RoutDocument
);
objApp = null;
}
}
/// <summary>
/// 循环合并多个文件(复制合并重复的文件)
/// </summary>
/// <param name="tempDoc">模板文件</param>
/// <param name="arrcopies">需要合并的文件</param>
/// <param name="outDoc">合并后的输出文件</param>
public void copyMerge(string tempDoc,string strcopyFolder,string outDoc)
{
string[] arrFiles = Directory.GetFiles(strcopyFolder);
copyMerge(tempDoc,arrFiles,outDoc);
}
#endregion
#region 循环合并多个文件(插入合并文件)
/// <summary>
/// 循环合并多个文件(插入合并文件)
/// </summary>
/// <param name="tempDoc">模板文件</param>
/// <param name="arrcopies">需要合并的文件</param>
/// <param name="outDoc">合并后的输出文件</param>
public void InsertMerge(string tempDoc,string outDoc)
{
object objMissing = Missing.Value;
object objFalse = false;
object confirmConversion = false;
object link = false;
object attachment = false;
try
{
//打开模板文件
Open(tempDoc);
foreach (string strcopy in arrcopies)
{
objApp.Selection.InsertFile(
strcopy,ref confirmConversion,ref link,ref attachment
);
}
//保存到输出文件
SaveAs(outDoc);
foreach (Document objDocument in objApp.Documents)
{
objDocument.Close(
ref objFalse,//OriginalFormat
ref objMissing //RoutDocument
);
objApp = null;
}
}
/// <summary>
/// 循环合并多个文件(插入合并文件)
/// </summary>
/// <param name="tempDoc">模板文件</param>
/// <param name="arrcopies">需要合并的文件</param>
/// <param name="outDoc">合并后的输出文件</param>
public void InsertMerge(string tempDoc,string outDoc)
{
string[] arrFiles = Directory.GetFiles(strcopyFolder);
InsertMerge(tempDoc,outDoc);
}
#endregion
}
}
相信本文所述对大家的C#程序设计有一定的借鉴价值。
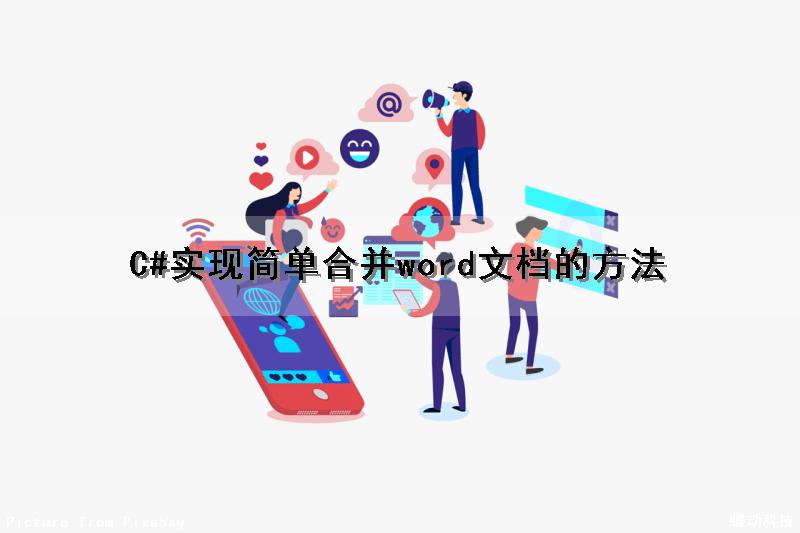
C#实现简单合并word文档的方法
本文实例讲述了C#实现简单合并word文档的方法。分享给大家供大家参考。具体如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Reflection;
namespace Demo
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
string path = @"C:\Documents and Settings\Administrator\桌面\output.doc";
string add = @"C:\Documents and Settings\Administrator\桌面\file";
private Microsoft.Office.Interop.Word.ApplicationClass applicationClass;
private Microsoft.Office.Interop.Word.Document doc;
private void button1_Click(object sender,EventArgs e)
{
Ex();
}
void Ex()
{
Open(path);
string[] files = System.IO.Directory.GetFiles(add);
foreach (string s in files)
{
InsertFile(s);
}
SaveAs(path);
}
/// <summary>
/// 打开输出word文档
/// </summary>
/// <param name="strFileName"></param>
public void Open(string strFileName)
{
applicationClass = new Microsoft.Office.Interop.Word.ApplicationClass();
object fileName = strFileName;
object readOnly = false;
object isVisible = true;
object missing = System.Reflection.Missing.Value;
doc = applicationClass.Documents.Open(ref fileName,ref missing,ref missing);
doc.Activate();
}
/// <summary>
/// 向打开的word文档中插入word文档
/// </summary>
/// <param name="strFileName"></param>
public void InsertFile(string strFileName)
{
object missing = System.Reflection.Missing.Value;
object confirmConversion = false;
object link = false;
object attachment = false;
applicationClass.Selection.InsertFile(strFileName,ref confirmConversion,ref link,ref attachment);
object pBreak = (int)Microsoft.Office.Interop.Word.WdBreakType.wdSectionBreakNextPage;
applicationClass.Selection.InsertBreak(ref pBreak);
}
/// <summary>
/// 最后保存word文档
/// </summary>
/// <param name="strFileName"></param>
public void SaveAs(string strFileName)
{
object missing = System.Reflection.Missing.Value;
object fileName = strFileName;
doc.SaveAs(ref fileName,ref missing);
}
}
}
@H_301_4@
希望本文所述对大家的C#程序设计有所帮助。
关于jsp实现针对excel及word文档的打印方法和用jsp方式打印出6×6表格的问题就给大家分享到这里,感谢你花时间阅读本站内容,更多关于asp.net(c#)下读取word文档的方法小结、C# 复制一个Word文档的部分或全部内容到另一个Word文档、C#实现合并多个word文档的方法、C#实现简单合并word文档的方法等相关知识的信息别忘了在本站进行查找喔。